Custom Errors
Welcome to tutorial no. 31 in our Golang tutorial series.
In the last tutorial we learnt about error representation in Go and how to handle errors from the standard library. We also learnt how to extract more information from the errors.
This tutorial deals with how to create our own custom errors which we can use in our functions and packages. We will also use the same techniques employed by the standard library to provide more details about our custom errors.
Creating custom errors using the New function
The simplest way to create a custom error is to use the New function of the errors package.
Before we use the New function to create a custom error, let’s understand how it is implemented. The implementation of the New function in the errors package is provided below.
1package errors
2
3// New returns an error that formats as the given text.
4// Each call to New returns a distinct error value even if the text is identical.
5func New(text string) error {
6 return &errorString{text}
7}
8
9// errorString is a trivial implementation of error.
10type errorString struct {
11 s string
12}
13
14func (e *errorString) Error() string {
15 return e.s
16}
The implementation is pretty simple. errorString
is a struct type with a single string field s
. The Error() string
method of the error
interface is implemented using a errorString
pointer receiver in line no. 14.
The New
function in line no. 5 takes a string
parameter, creates a value of type errorString
using that parameter and returns the address of it. Thus a new error is created and returned.
Now that we know how the New
function works, lets use it in a program of our own to create a custom error.
We will create a simple program which calculates the area of a circle and will return an error if the radius is negative.
1package main
2
3import (
4 "errors"
5 "fmt"
6 "math"
7)
8
9func circleArea(radius float64) (float64, error) {
10 if radius < 0 {
11 return 0, errors.New("Area calculation failed, radius is less than zero")
12 }
13 return math.Pi * radius * radius, nil
14}
15
16func main() {
17 radius := -20.0
18 area, err := circleArea(radius)
19 if err != nil {
20 fmt.Println(err)
21 return
22 }
23 fmt.Printf("Area of circle %0.2f", area)
24}
In the program above, we check whether the radius is less than zero in line no. 10. If so we return zero for the area along with the corresponding error message. If the radius is greater than 0, then the area is calculated and nil
is returned as the error in line no. 13.
In the main function, we check whether the error is not nil
in line no. 19. If it’s not nil
, we print the error and return, else the area of the circle is printed.
In this program the radius is less than zero and hence it will print,
Area calculation failed, radius is less than zero
Adding more information to the error using Errorf
The above program works well but wouldn’t it be nice if we print the actual radius which caused the error. This is where the Errorf function of the fmt package comes in handy. This function formats the error according to a format specifier and returns a string as value that satisfies the error
interface.
Let’s use the Errorf
function and make the program better.
1package main
2
3import (
4 "fmt"
5 "math"
6)
7
8func circleArea(radius float64) (float64, error) {
9 if radius < 0 {
10 return 0, fmt.Errorf("Area calculation failed, radius %0.2f is less than zero", radius)
11 }
12 return math.Pi * radius * radius, nil
13}
14
15func main() {
16 radius := -20.0
17 area, err := circleArea(radius)
18 if err != nil {
19 fmt.Println(err)
20 return
21 }
22 fmt.Printf("Area of circle %0.2f", area)
23}
In the program above, the Errorf
is used in line no. 10 to print the actual radius which caused the error. Running this program will output,
Area calculation failed, radius -20.00 is less than zero
Providing more information about the error using struct type and fields
It is also possible to use struct types which implement the error interface as errors. This gives us more flexibility with error handling. In our previous example, if we want to access the radius which caused the error, the only way now is to parse the error description Area calculation failed, radius -20.00 is less than zero
. This is not a proper way to do this since if the description changes, our code will break.
We will use the strategy followed by the standard library explained in the previous tutorial under the section “Converting the error to the underlying type and retrieving more information from the struct fields” and use struct fields to provide access to the radius which caused the error. We will create a struct type that implements the error interface and use its fields to provide more information about the error.
The first step would be create a struct type to represent the error. The naming convention for error types is that the name should end with the text Error
. So let’s name our struct type as areaError
1type areaError struct {
2 err string
3 radius float64
4}
The above struct type has a field radius
which stores the value of the radius responsible for the error and err
field stores the actual error message.
The next step is to implement the error interface.
1func (e *areaError) Error() string {
2 return fmt.Sprintf("radius %0.2f: %s", e.radius, e.err)
3}
In the above snippet, we implement the Error() string
method of the error interface using a pointer receiver *areaError
. This method prints the radius and the error description.
Let’s complete the program by writing the main
function and circleArea
function.
1package main
2
3import (
4 "errors"
5 "fmt"
6 "math"
7)
8
9type areaError struct {
10 err string
11 radius float64
12}
13
14func (e *areaError) Error() string {
15 return fmt.Sprintf("radius %0.2f: %s", e.radius, e.err)
16}
17
18func circleArea(radius float64) (float64, error) {
19 if radius < 0 {
20 return 0, &areaError{
21 err: "radius is negative",
22 radius: radius,
23 }
24 }
25 return math.Pi * radius * radius, nil
26}
27
28func main() {
29 radius := -20.0
30 area, err := circleArea(radius)
31 if err != nil {
32 var areaError *areaError
33 if errors.As(err, &areaError) {
34 fmt.Printf("Area calculation failed, radius %0.2f is less than zero", areaError.radius)
35 return
36 }
37 fmt.Println(err)
38 return
39 }
40 fmt.Printf("Area of rectangle %0.2f", area)
41}
In the program above, circleArea
in line no. 18 is used to calculate the area of the circle. This function first checks if the radius is less than zero, if so it creates a value of type areaError
using the radius responsible for the error and the corresponding error message and then returns the address of it in line no. 20 along with 0
as area. Thus we have provided more information about the error, in this case the radius which caused the error using the fields of a custom error struct.
If the radius is not negative, this function calculates and returns the area along with a nil
error in line no. 25.
In line no. 30 of the main function, we are trying to find the area of a circle with radius -20. Since the radius is less than zero, an error will be returned.
We check whether the error is not nil
in line no. 31 and in line no. 33 line we try to convert it to type *areaError
. If the error is of type *areaError
, we get the radius which caused the error in line no. 34 using areaError.radius
, print a custom error message and return from the program.
If the error is not of type *areaError
, we simply print the error in line no. 37 and return. If there is no error, the area will be printed in line no.40.
The program will print,
Area calculation failed, radius -20.00 is less than zero
Now lets use the second strategy described in the previous tutorial and use methods on custom error types to provide more information about the error.
Providing more information about the error using methods on struct types
In this section we will write a program which calculates the area of a rectangle. This program will print an error if either the length or width is less than zero.
The first step would be create a struct to represent the error.
1type areaError struct {
2 err string //error description
3 length float64 //length which caused the error
4 width float64 //width which caused the error
5}
The above error struct type contains an error description field along with the length and width which caused the error.
Now that we have the error type, lets implement the error interface and add a couple of methods on the error type to provide more information about the error.
1func (e *areaError) Error() string {
2 return e.err
3}
4
5func (e *areaError) lengthNegative() bool {
6 return e.length < 0
7}
8
9func (e *areaError) widthNegative() bool {
10 return e.width < 0
11}
In the above snippet, we return the description of the error from the Error() string
method. The lengthNegative() bool
method returns true when the length is less than zero and widthNegative() bool
method returns true when the width is less than zero. These two methods provide more information about the error, in this case they say whether the area calculation failed because of the length being negative or width being negative. Thus we have used methods on struct error types to provide more information about the error.
The next step is to write the area calculation function.
1func rectArea(length, width float64) (float64, error) {
2 err := ""
3 if length < 0 {
4 err += "length is less than zero"
5 }
6 if width < 0 {
7 if err == "" {
8 err = "width is less than zero"
9 } else {
10 err += ", width is less than zero"
11 }
12 }
13 if err != "" {
14 return 0, &areaError{
15 err: err,
16 length: length,
17 width: width,
18 }
19 }
20 return length * width, nil
21}
The rectArea
function above checks if either the length or width is less than zero, if so it returns an error of type *areaError
, else it returns the area of the rectangle with nil
as error.
Let’s finish this program by creating the main function.
1func main() {
2 length, width := -5.0, -9.0
3 area, err := rectArea(length, width)
4 if err != nil {
5 var areaError *areaError
6 if errors.As(err, &areaError) {
7 if areaError.lengthNegative() {
8 fmt.Printf("error: length %0.2f is less than zero\n", areaError.length)
9
10 }
11 if areaError.widthNegative() {
12 fmt.Printf("error: width %0.2f is less than zero\n", areaError.width)
13
14 }
15 return
16 }
17 fmt.Println(err)
18 return
19 }
20 fmt.Println("area of rect", area)
21}
In the main function, we check whether the error is not nil
in line no. 4. If it is not nil, we try to convert it to type *areaError
. Then using the lengthNegative()
and widthNegative()
methods, we check whether the error is because of the fact that the length is negative or width is negative. We print the corresponding error message and return from the program. Thus we have used the methods on the error struct type to provide more information about the error.
If there is no error, the area of the rectangle will be printed.
Here is the full program for your reference.
1package main
2
3import (
4 "errors"
5 "fmt"
6)
7
8type areaError struct {
9 err string //error description
10 length float64 //length which caused the error
11 width float64 //width which caused the error
12}
13
14func (e *areaError) Error() string {
15 return e.err
16}
17
18func (e *areaError) lengthNegative() bool {
19 return e.length < 0
20}
21
22func (e *areaError) widthNegative() bool {
23 return e.width < 0
24}
25
26func rectArea(length, width float64) (float64, error) {
27 err := ""
28 if length < 0 {
29 err += "length is less than zero"
30 }
31 if width < 0 {
32 if err == "" {
33 err = "width is less than zero"
34 } else {
35 err += ", width is less than zero"
36 }
37 }
38 if err != "" {
39 return 0, &areaError{
40 err: err,
41 length: length,
42 width: width,
43 }
44 }
45 return length * width, nil
46}
47
48func main() {
49 length, width := -5.0, -9.0
50 area, err := rectArea(length, width)
51 if err != nil {
52 var areaError *areaError
53 if errors.As(err, &areaError) {
54 if areaError.lengthNegative() {
55 fmt.Printf("error: length %0.2f is less than zero\n", areaError.length)
56
57 }
58 if areaError.widthNegative() {
59 fmt.Printf("error: width %0.2f is less than zero\n", areaError.width)
60
61 }
62 return
63 }
64 fmt.Println(err)
65 return
66 }
67 fmt.Println("area of rect", area)
68}
This program will print the output,
error: length -5.00 is less than zero
error: width -9.00 is less than zero
We have seen examples for two of the three ways described in the error handling tutorial to provide more information about the errors.
The third way using direct comparison is pretty straightforward. I would leave it as an exercise for you to figure out how to use this strategy to provide more information about our custom errors.
This brings us to an end of this tutorial.
Here is a quick recap of what we learnt in this tutorial,
- Creating custom errors using the New function
- Adding more information to the error using Errorf
- Providing more information about the error using struct type and fields
- Providing more information about the error using methods on struct types
I hope you liked this tutorial. Please leave your feedback and comments. Please consider sharing this tutorial on twitter and LinkedIn. Have a good day.
Next tutorial - Panic and Recover
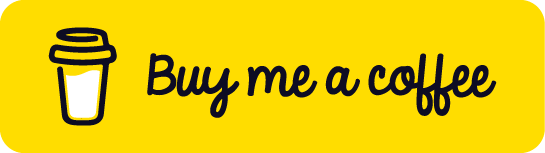