WebAssembly: DOM Access and Error Handling
Welcome to tutorial no. 2 of our WebAssembly tutorial series.
Series Index
Introduction to WebAssembly Using Go
Accessing DOM from Go using Javascript
In the first tutorial of this tutorial series, we created and exposed a function from Go and called it using JavaScript. I highly recommend reading the first part first part if you have not read it yet.
In this tutorial, we will develop a UI for our application, handle errors and also manipulate the DOM of the browser from Go.
Creating the UI and calling the wasm function
Let’s create a very simple UI using HTML. It will contain a text area to get the input JSON, a submit button to format the input JSON and another text area to display the output.
Let’s modify the existing ~/Documents/webassembly/assets/index.html
in the assets
folder to include the UI.
1<!doctype html>
2<html>
3 <head>
4 <meta charset="utf-8"/>
5 <script src="wasm_exec.js"></script>
6 <script>
7 const go = new Go();
8 WebAssembly.instantiateStreaming(fetch("json.wasm"), go.importObject).then((result) => {
9 go.run(result.instance);
10 });
11 </script>
12 </head>
13 <body>
14 <textarea id="jsoninput" name="jsoninput" cols="80" rows="20"></textarea>
15 <input id="button" type="submit" name="button" value="pretty json" onclick="json(jsoninput.value)"/>
16 <textarea id="jsonoutput" name="jsonoutput" cols="80" rows="20"></textarea>
17 </body>
18 <script>
19 var json = function(input) {
20 jsonoutput.value = formatJSON(input)
21 }
22 </script>
23</html>
In line no. 14 of the above HTML, we create a text area with id jsoninput
. This will be our text area where we input the JSON to be formatted.
Next, we create a submit button and when the button is clicked, the json
JavaScript function in line no. 19 will be called. This function takes the input JSON as a parameter, calls the formatJSON
wasm function which we created in the previous tutorial and sets the output to the jsonoutput
text area defined in line no. 16.
Let’s compile and run this program and see if it works.
1cd ~/Documents/webassembly/cmd/wasm/
2GOOS=js GOARCH=wasm go build -o ../../assets/json.wasm
3cd ~/Documents/webassembly/cmd/server/
4go run main.go
Go to the browser and type localhost:9090
. You can see the UI with two text areas and a button.
Input the following text in the first text area.
{"website":"golangbot.com", "tutorials": [{"title": "Strings", "url":"/strings/"}, {"title":"maps", "url":"/maps/"}, {"title": "goroutines","url":"/goroutines/"}]}
Now tap on the pretty json
button. You can see that the JSON is formatted and printed in the output text area.
You can see the above output in the browser. We have successfully called the wasm
function and formatted the JSON.
Accessing the DOM from Go using JavaScript
In the above section, we called the wasm
function, got the formatted JSON string output, and set the output text area with the formatted JSON using JavaScript.
There is one more way to achieve the same output. Instead of passing the formatted JSON string to javascript, it is possible to access the browser’s DOM from Go and set the formatted JSON string to the output text area.
Let’s see how this is done.
We need to modify the jsonWrapper
function in ~/Documents/webassembly/cmd/wasm/main.go
to achieve this.
1func jsonWrapper() js.Func {
2 jsonfunc := js.FuncOf(func(this js.Value, args []js.Value) any {
3 if len(args) != 1 {
4 return "Invalid no of arguments passed"
5 }
6 jsDoc := js.Global().Get("document")
7 if !jsDoc.Truthy() {
8 return "Unable to get document object"
9 }
10 jsonOuputTextArea := jsDoc.Call("getElementById", "jsonoutput")
11 if !jsonOuputTextArea.Truthy() {
12 return "Unable to get output text area"
13 }
14 inputJSON := args[0].String()
15 fmt.Printf("input %s\n", inputJSON)
16 pretty, err := prettyJson(inputJSON)
17 if err != nil {
18 errStr := fmt.Sprintf("unable to parse JSON. Error %s occurred\n", err)
19 return errStr
20 }
21 jsonOuputTextArea.Set("value", pretty)
22 return nil
23 })
24
25 return jsonfunc
26}
In line no. 6, we try to get the document
property of JavaScript from the global
scope. This property is needed to access the output JSON text area. The Truthy function in line no. 7 is JavaScript’s way of testing for nil
. If truthy returns false, it means the property doesn’t exist. Hence the appropriate error string is returned to JavaScript. We do not explicitly return a Go error type. The reason for this and how to handle errors is covered in the next section.
In line no. 10, we use the call method to call the getElementById
function on the jsDoc
JavaScript object and pass it the jsonoutput
argument. In JavaScript, this line of code corresponds to,
document.getElementById("jsonoutput");
If you recollect, jsonoutput
is the id
of the output text area in index.html
.
This will return the reference to the jsonoutput
text area. As we did earlier, we check for truthy
in the next line.
Now we have access to the jsonoutput
text area. In line no. 21, we use the Set method to set the value
property of the jsonoutput
text area to the formatted JSON string. The equivalent JavaScript function call is document.getElementById("jsonoutput").value = pretty
. This will display the formatted JSON in the output text area.
The changes to the Go side of the program is done.
A minor change is needed in the ~/Documents/webassembly/assets/index.html
. Since the JSON is set from Go directly by manipulating the browser’s DOM rather than JavaScript, we can remove the following piece of code.
Change line no. 20 from
jsonoutput.value = formatJSON(input)
to
var result = formatJSON(input)
console.log("Value returned from Go", result)
We have removed the code that sets jsonoutput
value from JavaScript since this is done from the Go side. We just log the result to the console. If there is an error in the JSON input, the error string that was returned from jsonfunc
will be logged to the console.
Do note that the output text area will not be cleared if there is an error. It will still keep showing its existing content. This will be fixed in the next section.
Try running the program again using the following commands and then opening localhost:9090
in the browser.
cd ~/Documents/webassembly/cmd/wasm/
GOOS=js GOARCH=wasm go build -o ../../assets/json.wasm
cd ~/Documents/webassembly/cmd/server/
go run main.go
The output will be the same. If a Valid JSON is passed, it will be formatted and printed. This is now done from the Go code by manipulating the DOM rather than from the JavaScript. If you pass an invalid JSON, the corresponding error will be logged to the console.
Error Handling
In the previous section, we just returned a string from the jsonfunc
function when an error occurred during the JSON formatting.
The idiomatic way of handling errors in Go is to return the error
. Let’s modify the jsonWrapper
function in ~/Documents/webassembly/cmd/wasm/main.go
to return an error and see what happens.
1func jsonWrapper() js.Func {
2 jsonfunc := js.FuncOf(func(this js.Value, args []js.Value) any {
3 if len(args) != 1 {
4 return errors.New("Invalid no of arguments passed")
5 }
6 jsDoc := js.Global().Get("document")
7 if !jsDoc.Truthy() {
8 return errors.New("Unable to get document object")
9 }
10 jsonOuputTextArea := jsDoc.Call("getElementById", "jsonoutput")
11 if !jsonOuputTextArea.Truthy() {
12 return errors.New("Unable to get output text area")
13 }
14 inputJSON := args[0].String()
15 fmt.Printf("input %s\n", inputJSON)
16 pretty, err := prettyJson(inputJSON)
17 if err != nil {
18 errStr := fmt.Sprintf("unable to parse JSON. Error %s occurred\n", err)
19 return errors.New(errStr)
20 }
21 jsonOuputTextArea.Set("value", pretty)
22 return nil
23 })
24 return jsonfunc
25}
Line no. 4 is changed to return an error
instead of a string
. Similar changes are done in other places where error needs to be returned.
Compile and run the code and try inputting an incorrect JSON and see what happens. I have provided the invalid JSON string dfs333{"website
as input.
The program has crashed with the following stack trace.
input dfs333{"website wasm_exec.js:22:14
panic: ValueOf: invalid value wasm_exec.js:22:14
<empty string> wasm_exec.js:22:14
goroutine 6 [running]: wasm_exec.js:22:14
syscall/js.ValueOf({0x12120, 0x40e3a0}) wasm_exec.js:22:14
/usr/local/go/src/syscall/js/js.go:208 +0xf7 wasm_exec.js:22:14
syscall/js.Value.Set({{}, 0x7ff8000100000012, 0x4100b0}, {0x242f1, 0x6}, {0x12120, 0x40e3a0}) wasm_exec.js:22:14
/usr/local/go/src/syscall/js/js.go:303 +0x8 wasm_exec.js:22:14
syscall/js.handleEvent() wasm_exec.js:22:14
/usr/local/go/src/syscall/js/func.go:95 +0x27 wasm_exec.js:22:14
exit code: 2 wasm_exec.js:101:14
Value returned from Go undefined localhost:9090:20:21
As we already discussed in the last tutorial, any value returned by jsonfunc
will automatically be mapped to the corresponding JavaScript value using the ValueOf function. If you take a quick look at the documentation of this function, you can see that there is no mapping for Go’s error
type to a corresponding JavaScript type. This is the reason the program is crashing with error panic: ValueOf: invalid value
when an error
type is returned from Go. There is no way to pass errors from Go to Javascript currently. This feature could be added in the future, but currently, it’s not available. We have to look at other options when returning errors.
One way to do this is to establish a contract between Go and JavaScript. For example, we can return a map from Go to JavaScript. If the map contains an error
key, it can be considered as an error by JavaScript and handled appropriately.
Let’s modify the jsonWrapper
function to do this.
1func jsonWrapper() js.Func {
2 jsonfunc := js.FuncOf(func(this js.Value, args []js.Value) any {
3 if len(args) != 1 {
4 result := map[string]any{
5 "error": "Invalid no of arguments passed",
6 }
7 return result
8 }
9 jsDoc := js.Global().Get("document")
10 if !jsDoc.Truthy() {
11 result := map[string]any{
12 "error": "Unable to get document object",
13 }
14 return result
15 }
16 jsonOuputTextArea := jsDoc.Call("getElementById", "jsonoutput")
17 if !jsonOuputTextArea.Truthy() {
18 result := map[string]any{
19 "error": "Unable to get output text area",
20 }
21 return result
22 }
23 inputJSON := args[0].String()
24 fmt.Printf("input %s\n", inputJSON)
25 pretty, err := prettyJson(inputJSON)
26 if err != nil {
27 errStr := fmt.Sprintf("unable to parse JSON. Error %s occurred\n", err)
28 result := map[string]any{
29 "error": errStr,
30 }
31 return result
32 }
33 jsonOuputTextArea.Set("value", pretty)
34 return nil
35 })
36 return jsonfunc
37}
In the above snippet, in line no. 4, a map named result
with an error
key is created and returned with the corresponding error. Similar changes are done in other places. The JavaScript side can now check for the existence of this key. If this key is present, it means an error has occurred and it can be handled appropriately.
The modified index.html
file is provided below. Changes are done only to the JavaScript section starting at line no. 18.
1...
2 <script>
3 var json = function(input) {
4 var result = formatJSON(input)
5 if (( result != null) && ('error' in result)) {
6 console.log("Go return value", result)
7 jsonoutput.value = ""
8 alert(result.error)
9 }
10 }
11 </script>
12</html>
The return value from Go is first validated for null
and then it is checked to find whether the error
key is present. If the error key is present, it means some error has occurred when processing the JSON. The output text area is first cleared and then a popup alert is shown to the user with the error message.
Compile and run the program again. Try passing an invalid JSON. You can see an alert with the error message. The output text area is also cleared.
One more question might popup in your mind. Why are we using a map[string]any
instead of a map[string]string
. The reason is the same as we discussed earlier. If you take a quick look at the documentation of the ValueOf function, you can see that there is no mapping for map[string]string
from Go to JavaScript. Hence we use map[string]any
, the type alias of map[string]interface{}
which has a corresponding mapping in JavaScript.
This brings us to the end of this tutorial.
The source code is available at https://github.com/golangbot/webassembly/tree/tutorial2
I hope you liked this tutorial. Please leave your feedback and comments. Please consider sharing this tutorial on twitter or LinkedIn. Have a good day.
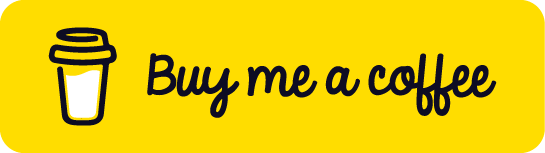