June 2019 Quiz Answers and Explanation
Here are all the quizzes posted on Twitter and Facebook in June 2019 and their explanation.
Quiz 1
1package main
2
3import (
4 "fmt"
5)
6
7func hello() []string {
8 return nil
9}
10
11func main() {
12 h := hello
13 if h == nil {
14 fmt.Println("nil")
15 } else {
16 fmt.Println("not nil")
17 }
18}
Options
nil
not nil
compilation error
Answer
not nil
We assign the function hello
to the variable h
and not the return value of hello()
in line no. 12 and hence h
is not nil and the program will print not nil
.
Quiz 2
1package main
2
3import (
4 "fmt"
5 "strconv"
6)
7
8func main() {
9 i := 2
10 s := "1000"
11 if len(s) > 1 {
12 i, _ := strconv.Atoi(s)
13 i = i + 5
14 }
15 fmt.Println(i)
16}
Options
2
1005
compilation error
Answer
2
The tricky part of the above quiz is line no. 12. i, _ := strconv.Atoi(s)
creates a new variable i
whose scope is only within the if
statement. The i
which is being printed in line no. 15 is actually the one defined in line no. 9 and not the one defined in line no. 12. Hence this program will print 2
.
Quiz 3
1package main
2
3import (
4 "fmt"
5)
6
7func hello(num ...int) {
8 num[0] = 18
9}
10
11func main() {
12 i := []int{5, 6, 7}
13 hello(i...)
14 fmt.Println(i[0])
15}
Options
18
5
Compilation error
Answer
18
The slice i
is passed to the variadic function hello()
function in line no. 13. To know why this happens, please read the section Passing a slice to a variadic function in /variadic-functions/.
If you read the section Passing a slice to a function in /arrays-and-slices/, you can understand that changes made to a slice inside a function are visible to the caller. Hence line no. 14 will print 18
.
Quiz 4
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 a := [2]int{5, 6}
9 b := [2]int{5, 6}
10 if a == b {
11 fmt.Println("equal")
12 } else {
13 fmt.Println("not equal")
14 }
15}
Options
compilation error
equal
not equal
Answer
equal
Arrays are value types in Go and can be compared. Two array values are equal if their corresponding elements are equal. In our case, a
and b
are equal and hence this program prints equal
.
Quiz 5
1package main
2
3import "fmt"
4
5type rect struct {
6 len, wid int
7}
8
9func (r rect) area() {
10 fmt.Println(r.len * r.wid)
11}
12
13func main() {
14 r := &rect{len: 5, wid: 6}
15 r.area()
16}
Options
compilation error
30
Answer
30
This program will compile perfectly and print 30
.
In line no. 14 of the program above, we assign the address of rect
to r
. You might be wondering why the program worked when we didn’t use (*r).area()
in line no. 15. Since area()
has a value receiver, Go is intelligent enough to interpret r.area()
as (*r).area()
and hence this program works :).
Quiz 6
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 a := [5]int{1, 2, 3, 4, 5}
9 t := a[3:4:4]
10 fmt.Println(t[0])
11}
Options
3
4
compilation error
Answer
4
The expression
a[low : high : max]
constructs a slice of the same type, and with the same length and elements as the simple slice expression a[low : high]. Additionally, it controls the resulting slice’s capacity by setting it to max - low. Hence, the slice t
in line no.9 has one element 4
and is of capacity 1
.
Quiz 7
1package main
2
3import (
4 "fmt"
5)
6
7type person struct {
8 name string
9}
10
11func main() {
12 var m map[person]int
13 p := person{"mike"}
14 fmt.Println(m[p])
15}
Options
compilation error
0
1
Answer
0
When we try to print an element that does not exist in a map, the zero value of the element is printed. In our case m
is a map of type map[person]int
. Since p
doesn’t exist in the map, the zero value of int i.e 0
is printed.
Quiz 8
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 i := 65
9 fmt.Println(string(i))
10}
Options
A
65
compilation error
Answer
A
The unicode value of A
is 65
. Hence when i
is type casted to string in line no. 9, A
is printed.
Quiz 9
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 a := 5
9 b := 8.1
10 fmt.Println(a + b)
11}
Options
13.1
13
compilation error
Answer
compilation error
a is of type int
and b is of type float64
. We are trying to add a int
and float64
in line no. 10. This is not allowed and hence the program will fail to compile with error ./prog.go:10:16: invalid operation: a + b (mismatched types int and float64)
Quiz 10
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 var i interface{}
9 if i == nil {
10 fmt.Println("nil")
11 return
12 }
13 fmt.Println("not nil")
14}
Options
nil
not nil
compilation error
Answer
nil
An empty interface has both its underlying value and concrete type as nil
. Hence i
is nil
.
Quiz 11
1package main
2
3import (
4 "fmt"
5)
6
7func hello(i int) {
8 fmt.Println(i)
9}
10func main() {
11 i := 5
12 defer hello(i)
13 i = i + 10
14}
Options
5
15
Answer
5
The arguments of a deferred function are evaluated when the defer statement is executed and not when the actual function call is done. Hence when the defer statement is encountered in line no. 12, the value of i
is 5. So this program will print 5
.
Quiz 12
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 fmt.Printf("%%")
9}
Options
0.0
compilation error
%
Answer
%
The format specifier %%
prints a literal %
sign. Hence the program prints %
.
Quiz 13
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 s := make(map[string]int)
9 delete(s, "h")
10 fmt.Println(s["h"])
11}
Options
runtime panic
0
compilation error
Answer
0
The delete function in line no. 9 doesn’t return anything and will do nothing if the specified key doesn’t exist. Since the key h
doesn’t exist, the delete function will not do anything. In line no. 10, we are trying to print s["h"]
. Since the map s
doesn’t have the key h
, it will return the default value of int
. Hence 0
will be printed.
Quiz 14
1package main
2
3import (
4 "fmt"
5)
6
7func main() {
8 i := -5
9 j := +5
10 fmt.Printf("%+d %+d", i, j)
11}
Options
-5 +5
+5 +5
0 0
Answer
-5 +5
The +
flag in the format specifier %+d
is used to always print a sign for numeric values. Hence this program outputs -5 +5
.
Like my tutorials? Please show your support by donating. Your donations will help me create more awesome tutorials.
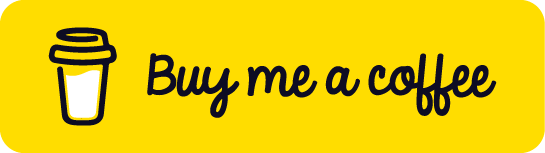